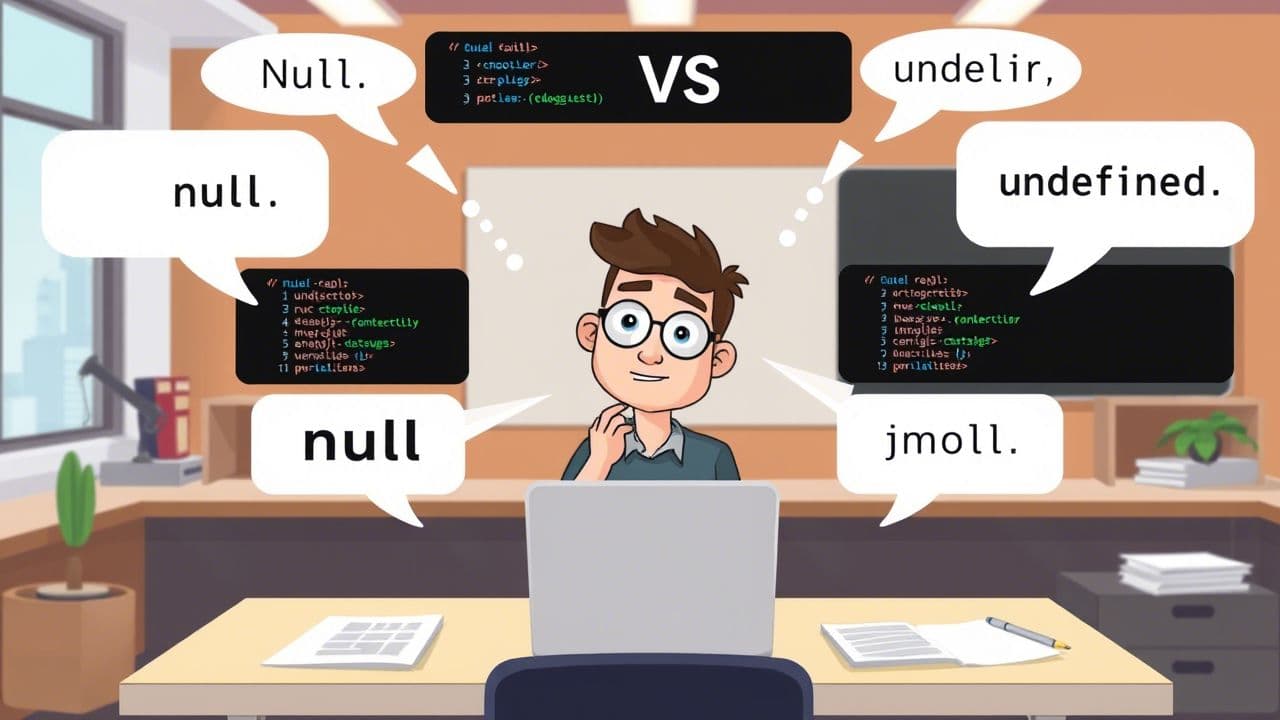
dev Await
February 2025
JavaScript Null vs Undefined: The Essential Guide
What are null and undefined? 🤔
Undefined
Represents a variable that has been declared but not assigned a value
Default value of function parameters
Function return value when nothing is returned
Null
Represents intentional absence of any object value
Must be explicitly assigned
Is an object type (a JavaScript quirk)
Key Differences 📝
// Undefined examples
let name;
console.log(name); // undefined
function greet() {
// no return statement
}
console.log(greet()); // undefined
// Null examples
let user = null;
console.log(user); // null
let settings = {
theme: null // explicitly set to null
};
Common Scenarios 🎯
Function Parameters
function displayUser(name = 'Guest') { console.log(name); } displayUser(); // 'Guest' displayUser(undefined); // 'Guest' displayUser(null); // null
Object Properties
const user = { name: 'John', age: null, // explicitly no age }; console.log(user.name); // 'John' console.log(user.age); // null console.log(user.email); // undefined (property doesn't exist)
Best Practices 💡
// Checking for both null and undefined const isNullOrUndefined = (value) => value == null; // Default values const name = username ?? 'Guest'; // Nullish coalescing const age = user.age || 0; // Logical OR // Optional chaining const userCity = user?.address?.city; // Safe property access
Common Mistakes to Avoid ⚠️
Not Using Strict Equality
// Bad if (value == null) { } // Good if (value === null) { } if (value === undefined) { }
Unnecessary Null Checks
// Unnecessary if (value !== null && value !== undefined) { } // Better if (value != null) { }
Best Practices 🌟
Use undefined for unintentional missing values
Use null for intentional missing values
Use optional chaining (?.) for safe property access
Use nullish coalescing (??) for default values
Be explicit in function returns
Quick Summary 📌
undefined
is assigned by JavaScriptnull
is assigned by developersBoth represent "no value"
typeof null
is "object"typeof undefined
is "undefined"Use strict equality (
===
) for type-safe comparisons